Creating Python programs that simulate the action of each Day of Creation according to the narrative found in Genesis can provide an interesting way to engage with these biblical accounts. Here’s how you might conceive of these seven “Day” programs in Python, taking a bit of creative liberty to connect programming concepts with the biblical text.
These Python programs honor the essence of the creation narratives found in Genesis, celebrating the act of creation through code. They serve as a creative way to intertwine faith with technology, reflecting on the profound work of creation told in the Bible.
Integrating Faith with Technology Through Python Programming
Day 1 – Creation of Light
def create_light():
light_status = "off"
print("And God said, 'Let there be light'")
light_status = "on"
print("And there was light.")
return light_status
light_status = create_light()
print(f"Light status: {light_status}")
Day 2 – Creation of Sky (Firmament)
def create_sky():
waters = ["waters below", "waters above"]
sky = "firmament in the midst of the waters"
print("And God made the firmament")
waters.remove("waters above") # This simplifies our example, assuming the action of dividing waters.
print(f"The waters are now {waters} and sky is called {sky}")
return sky
sky = create_sky()
print(f"Sky: {sky}")
Day 3 – Creation of Land and Vegetation
def create_land_and_vegetation():
earth = {"dry land": "Earth", "gathering waters": "Seas"}
vegetation = ["grass", "herb yielding seed", "fruit tree yielding fruit"]
print("And God called the dry land Earth; and the gathering together of the waters called he Seas.")
print("And the earth brought forth vegetation.")
return earth, vegetation
earth, vegetation = create_land_and_vegetation()
print(f"Earth: {earth}, Vegetation: {vegetation}")
Day 4 – Creation of Celestial Bodies
def create_celestial_bodies():
celestial_bodies = {"greater light": "Sun", "lesser light": "Moon", "stars": "Stars"}
print("And God made two great lights; the greater light to rule the day, and the lesser light to rule the night.")
return celestial_bodies
celestial_bodies = create_celestial_bodies()
print(f"Celestial Bodies: {celestial_bodies}")
Day 5 – Creation of Marine Life and Birds
def create_marine_life_and_birds():
marine_life = ["great whales", "every living creature in the waters"]
birds = ["every winged fowl"]
print("And God created great whales, and every living creature that moveth in the waters.")
return marine_life, birds
marine_life, birds = create_marine_life_and_birds()
print(f"Marine Life: {marine_life}, Birds: {birds}")
Day 6 – Creation of Land Animals and Humans
def create_land_animals_and_humans():
animals = ["cattle", "creeping thing", "beast of the earth"]
humans = "man in our image"
print("And God made the beast of the earth, and cattle, and every thing that creepeth upon the earth.")
print("And God said, Let us make man in our image.")
return animals, humans
animals, humans = create_land_animals_and_humans()
print(f"Land Animals: {animals}, Humans: {humans}")
Day 7 – Rest
def rest():
print("And on the seventh day God ended his work which he had made; and he rested on the seventh day.")
work_done = True
return work_done
rest_status = rest()
print(f"God rested: {rest_status}")
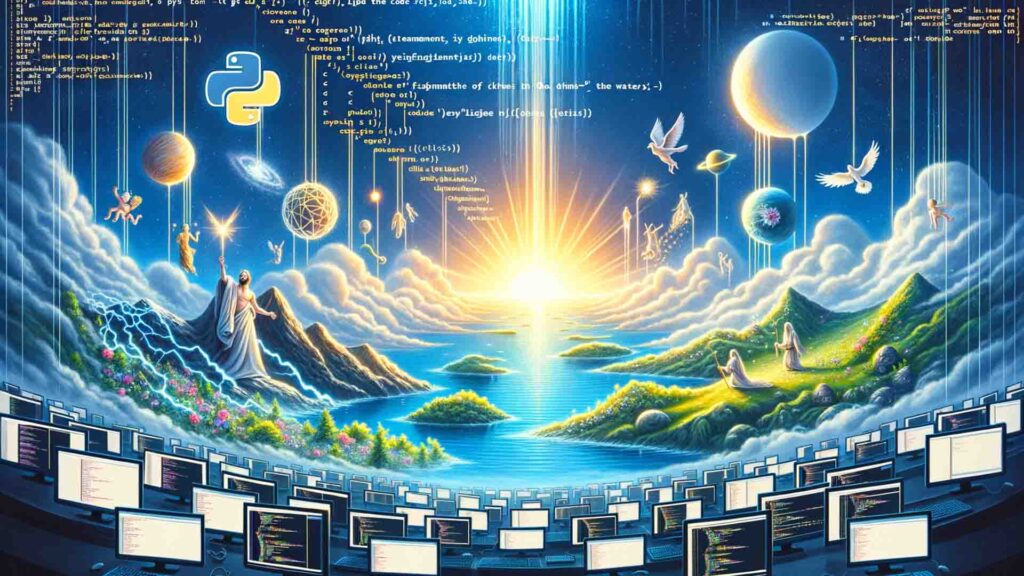
Code with an understanding of loops in programming
# Program to simulate the 7 Days of Creation according to the Bible
days_of_creation = {
1: "God created light, separating light from darkness.",
2: "God created the firmament and separated the waters.",
3: "God gathered waters to reveal dry land and brought forth vegetation.",
4: "God created the sun, moon, and stars to govern time and give light.",
5: "God filled the waters with sea creatures and the skies with birds.",
6: "God created land animals and humans in His own image.",
7: "God rested, blessing the seventh day and making it holy."
}
def simulate_creation(days):
for day, action in days.items():
print(f"Day {day}: {action}")
if day < 7: # Rest only occurs on the seventh day
print("And God saw it was good.")
elif day == 7:
print("And God rested from all His work.")
print("And the evening and the morning were the", ordinal(day), "day.\n")
def ordinal(n):
# Converts number to its ordinal representation
return "%d%s" % (n, "tsnrhtdd"[(n//10%10!=1)*(n%10<4)*n%10::4])
simulate_creation(days_of_creation)
Code with an understanding of objects and classes in programming
class Creation:
def __init__(self):
self.light_status = "off"
self.firmament = "none"
self.land_and_water_defined = False
self.vegetation = []
self.celestial_bodies = []
self.marine_life_and_birds = []
self.land_animals_and_humans = []
self.rest_day = False
def day_one(self):
print("Day 1: Creation of light.")
self.light_status = "on"
print("Light is now:", self.light_status)
def day_two(self):
print("\nDay 2: Creation of sky.")
self.firmament = "created"
print("The firmament is now:", self.firmament)
def day_three(self):
print("\nDay 3: Creation of land and vegetation.")
self.land_and_water_defined = True
self.vegetation = ["grass", "herb yielding seed", "fruit tree yielding fruit"]
print("Land defined:", self.land_and_water_defined)
print("Vegetation created:", ', '.join(self.vegetation))
def day_four(self):
print("\nDay 4: Creation of celestial bodies.")
self.celestial_bodies = ['Sun', 'Moon', 'Stars']
print("Celestial bodies created:", ', '.join(self.celestial_bodies))
def day_five(self):
print("\nDay 5: Creation of marine life and birds.")
self.marine_life_and_birds = ["great whales", "every living creature in the waters", "winged fowl"]
print("Marine life and birds created:", ', '.join(self.marine_life_and_birds))
def day_six(self):
print("\nDay 6: Creation of land animals and humans.")
self.land_animals_and_humans = ["cattle", "creeping thing", "beast of the earth", "humans"]
print("Land animals and humans created:", ', '.join(self.land_animals_and_humans))
def day_seven(self):
print("\nDay 7: God rested.")
self.rest_day = True
print("God rested:", self.rest_day)
world = Creation()
world.day_one()
world.day_two()
world.day_three()
world.day_four()
world.day_five()
world.day_six()
world.day_seven()